Well, today I finally start evaluating Visual Studio Team System. Yesterday I upgraded my PC to 2 Gig of RAM as the Virtual PC image with VSTS beta 2 requires 1.5 Gig on it's own.
I am excited about the new version control system (SCC) mainly because having worked with Visual Source Safe in previous projects, I am all too aware of the need for a decent offering from Microsoft in the Source Control department, although I am going to be quite hard to please given that I have been using Subversion for my own projects for some time now, and have found it to be very good.
I am also interested in the unit testing and code coverage modules.
I have to confess though, I don't think the productivity gains are going to be quite as dramatic as the Microsoft hype is making out. The best that we can hope for is that with the tighter integration of all these aspects of the SDLC, there will be a small time saving in people not having to switch between applications to perform development related tasks, and that reporting on project status will become easier.
There are a few reasons I believe this.
- A good, productive software development team will already have processes in place that suit there projects, their tool set and their team structure, they will already have worked out procedures around the fact that their tools aren't completely integrated, so the integration of the tools can save a small amount of time here.
- A good team will also be disciplined in following procedure, If a code review or static code analysis is required before checkin, a disciplined team will adhere to this, and do not really need software to tell them that they've forgotten to write unit tests. In this case the only benefit I can see is when new staff are introduced to the development cycle, the procedures will be more evident and they may learn them quicker.
- A good development team will have good leadership that will keep an eye on the processes tweaking them if there are issues with the code produced, or if the procedures are becoming too burdensome.
- A bad development team would have discipline problems that require more than just some software rules to solve. In my experience, where there is a lack of discipline, it is either out of laziness, or a lack of understanding of the reasons behind the procedures and policies. Laziness is a serious problem, however a lack of understanding can be solved only through education and experience.
- A good team will have communications in place. One presentation I've seen suggests that with VSTS there will be no more need for weekly status meetings because the work item tracking and the automatic reporting will be enough to display status. Maybe the actual status reporting in such meetings could be simplified, but I have always found these kinds of meetings vital as they provide a forum for everybody, (not just the project manager) to get a feel for what is going on across the entire project, so developer X might be complaining about a problem he's having and developer Y says that he has just finished some code that solves a similar problem, and would just require some re-factoring to solve his particular issue, while tester A is struggling with a particular concept in the use cases, so one of the BA's offers to explain it a bit more thoroughly for the entire team who then suggest she re-word the use case slightly. These kinds of informal meetings are invaluable to a dev team, and until we start writing software with advanced AI, they cannot be replaced by software.
Anyway, I still think VSTS will be a worthwhile tool, but I just think we need to be realistic about how far software tools can take us, and we need to not under estimate the human side of software development.
Sunday, July 17, 2005
Sunday, July 10, 2005
The code wars have begun
Fellow collegues Mitch Denny and Joseph Cooney have started the "Iron Coder" challenge over the question is it better to sub-class or use extender providers. It will be a very interesting battle, with dark lord Darth Denny defending extender providers, and the young Jedi Joseph upholding the cause for sub-classing.
Use the source young Jedi.
Use the source young Jedi.
Friday, July 08, 2005
Great use for nested classes
When I first read about nested classes I thought it was just one of those obscure parts of c# that I was never likely to find a decent use for, but today I found quite a good use for it, and it was sitting right there in my previous post.
Usually we use a custom control to contain an UltraGrid like so
In my last post I offered a solution to adding record headers to an UltraGrid, but I passed the RowHeaders in as a List parameter to the constructor, now in the problem I'm trying to solve, this list is already maintained by the user control, so it means I have to maintain in 2 separate places..... Well not any more
Then at the business end of things in DrawElement I use the following
This means that I can let the user control maintain the row names and no-one ever needs to know about our RowSelectorDrawFilter class as it's happily nested in MyControlClass.
Usually we use a custom control to contain an UltraGrid like so
public class MyControl : UserControl
{
...
private UltraGrid myGrid;
}
In my last post I offered a solution to adding record headers to an UltraGrid, but I passed the RowHeaders in as a List
public class MyControl : UserControl
{
...
private list myRowNames;
private UltraGrid myGrid;
private class RowSelectorDrawFilter : IUIElementDrawFilter
{
private RowSelectorDrawFilter(MyControl control)
{
myControl = control;
}
...
private MyControl myControl;
}
}
Then at the business end of things in DrawElement I use the following
...
drawParams.DrawString(drawParams.Element.Rect, myControl.myRowNames[row.ListIndex], false, false);
...
This means that I can let the user control maintain the row names and no-one ever needs to know about our RowSelectorDrawFilter class as it's happily nested in MyControlClass.
Saturday, July 02, 2005
Row Headers in infragistics UltraGrid control
A problem was puzzling me with the infragistics UltraGrid control the other day, how to get row headers in the grid?
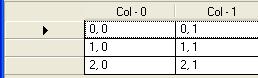
My first thought was "well the UltraGrid is such a huge control, there must be some property somewhere I can set to do this", something like.... myGrid.DisplayLayout.Rows[i].Header = "Row Name" or myGrid.DisplayLayout.Override.RowSelectors[i].Text = "Row Name" etc.... I spent ages looking all to no avail.
After all else failed, I resorted (as a last ditch attempt) to the infragistics knowledge base where I found this article.
Now this just talks about getting rid of the pencil, but slightly adapt it and you get the following
then add the following line to the OnLoad method
and providing the row names list is in the same order as the underlying table, it all just works. It is important that row.ListIndex is used to index into the rowNames array instead of row.Index, as row.Index is the display index, and if the grid is sorted, the row names will not be sorted.
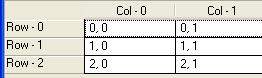
Hope this helps someone else out there.
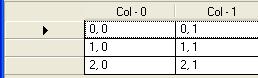
My first thought was "well the UltraGrid is such a huge control, there must be some property somewhere I can set to do this", something like.... myGrid.DisplayLayout.Rows[i].Header = "Row Name" or myGrid.DisplayLayout.Override.RowSelectors[i].Text = "Row Name" etc.... I spent ages looking all to no avail.
After all else failed, I resorted (as a last ditch attempt) to the infragistics knowledge base where I found this article.
Now this just talks about getting rid of the pencil, but slightly adapt it and you get the following
class RowSelectorDrawFilter : IUIElementDrawFilter
{
public RowSelectorDrawFilter(List<string> rowNames)
{
myRowNames = rowNames;
}
public DrawPhase GetPhasesToFilter(ref UIElementDrawParams drawParams)
{
// Drawing RowSelector Call DrawElement Before the Image is Drawn
if (drawParams.Element is Infragistics.Win.UltraWinGrid.RowSelectorUIElement)
return DrawPhase.BeforeDrawImage;
else
return DrawPhase.None;
}
public bool DrawElement(DrawPhase drawPhase, ref UIElementDrawParams drawParams)
{
// If the image isn't drawn yet, and the UIElement is a RowSelector
if (drawPhase == DrawPhase.BeforeDrawImage && drawParams.Element is Infragistics.Win.UltraWinGrid.RowSelectorUIElement)
{
// Get a handle of the row that is being drawn
UltraGridRow row = (UltraGridRow)drawParams.Element.GetContext(typeof(UltraGridRow));
drawParams.DrawString(drawParams.Element.Rect, myRowNames[row.ListIndex], false, false);
return true;
}
// Else return false, to draw as normal
return false;
}
private List<string> myRowNames = null;
}
then add the following line to the OnLoad method
myTableGrid.DrawFilter = new RowSelectorDrawFilter(myRowNames);
and providing the row names list is in the same order as the underlying table, it all just works. It is important that row.ListIndex is used to index into the rowNames array instead of row.Index, as row.Index is the display index, and if the grid is sorted, the row names will not be sorted.
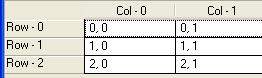
Hope this helps someone else out there.
Subscribe to:
Posts (Atom)